Modern Loop
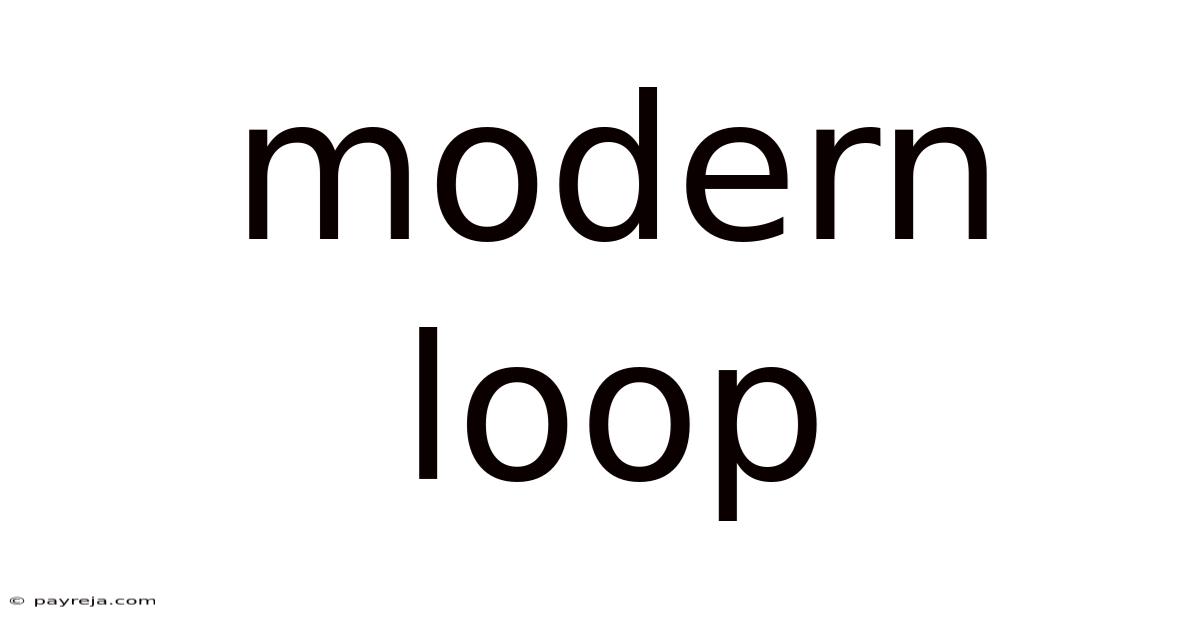
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit Best Website meltwatermedia.ca. Don't miss out!
Table of Contents
Mastering the Modern Loop: A Deep Dive into Iterative Programming Techniques
What if mastering iterative programming could unlock the power of efficient and elegant code? Modern looping techniques are revolutionizing software development, paving the way for cleaner, more maintainable, and performant applications.
Editor’s Note: This article on modern looping techniques was published today, offering the latest insights into efficient iterative programming practices.
The humble loop, a fundamental building block of programming, has undergone a significant evolution. While traditional for
and while
loops remain essential, modern programming paradigms have introduced refined approaches that enhance code readability, maintainability, and performance. Understanding and effectively utilizing these modern looping techniques is crucial for any developer aiming to write elegant, efficient, and scalable code. This article explores the core concepts of modern loops, examining various techniques and highlighting their applications across different programming languages and contexts.
This article will cover the following key areas: the evolution of looping constructs, a comparison of traditional and modern approaches, an in-depth analysis of iterator-based loops, the use of higher-order functions for iteration, handling exceptions within loops, and best practices for choosing the right looping technique. You will gain a comprehensive understanding of modern looping techniques, empowering you to write more efficient and maintainable code.
The Evolution of Looping Constructs
The history of loops is deeply intertwined with the development of programming languages themselves. Early languages often relied on rudimentary goto
statements to create iterative processes, leading to notoriously difficult-to-read and maintain "spaghetti code." The introduction of structured programming paradigms brought about the for
and while
loops, promoting cleaner and more organized code. However, even these constructs can become cumbersome and difficult to understand in complex scenarios.
Modern languages have responded to this challenge by introducing more sophisticated looping mechanisms, often leveraging iterator patterns and higher-order functions. These advancements aim to improve code readability, reduce boilerplate code, and enhance performance.
Traditional Loops vs. Modern Approaches
Traditional for
and while
loops, while effective for simple iterations, can become unwieldy when dealing with complex data structures or intricate logic. For instance, iterating over a nested array using nested for
loops can quickly lead to deeply nested and confusing code. Modern approaches often simplify these scenarios, enhancing both readability and efficiency.
Consider the task of summing the elements of an array. A traditional for
loop approach might look like this (in JavaScript):
let array = [1, 2, 3, 4, 5];
let sum = 0;
for (let i = 0; i < array.length; i++) {
sum += array[i];
}
console.log(sum); // Output: 15
A more modern approach using array methods (also in JavaScript) would be:
let array = [1, 2, 3, 4, 5];
let sum = array.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
console.log(sum); // Output: 15
The second example is significantly more concise and arguably more readable. The reduce
method encapsulates the iteration logic, making the code cleaner and easier to understand.
Iterator-Based Loops
Iterator patterns provide a powerful and elegant approach to iteration. An iterator is an object that defines a sequence and a method to traverse that sequence. Many modern languages incorporate iterators into their core libraries, making them readily available for various data structures.
Python, for example, provides iterators through its built-in functions and its support for iterable objects. The for
loop in Python implicitly utilizes iterators:
my_list = [1, 2, 3, 4, 5]
for item in my_list:
print(item)
This concise syntax hides the underlying iterator mechanism, making the code easy to read and write.
Higher-Order Functions for Iteration
Higher-order functions (functions that take other functions as arguments or return them) provide a powerful tool for expressing iteration in a functional programming style. Languages like JavaScript, Python, and others offer a range of higher-order functions specifically designed for iteration, such as map
, filter
, and reduce
.
These functions allow you to express iterative operations in a more declarative way, focusing on what needs to be done rather than how it should be done. This leads to more concise and often more efficient code.
Handling Exceptions Within Loops
When working with loops, it's crucial to handle potential exceptions gracefully. For example, if you're iterating over a file, an error might occur if the file is not found or becomes corrupted. Modern languages provide robust exception-handling mechanisms (like try-except
blocks in Python or try-catch
blocks in JavaScript) to handle these scenarios without causing the entire program to crash. Proper exception handling is crucial for creating robust and reliable software.
Choosing the Right Looping Technique
Selecting the appropriate looping technique depends on various factors, including the complexity of the iteration, the data structure being traversed, and the overall coding style. For simple iterations over arrays or lists, traditional for
loops might suffice. However, for more complex scenarios, iterator-based loops or higher-order functions often provide a more elegant and efficient solution. The key is to prioritize readability and maintainability, selecting the technique that best suits the specific task.
The Connection Between Concurrency and Modern Loops
Concurrency, the ability to execute multiple tasks seemingly simultaneously, is becoming increasingly important in modern software development. Traditional loops often struggle with concurrency, as they typically execute sequentially. However, modern approaches, particularly those using iterators and functional programming paradigms, often lend themselves more readily to concurrent execution. Libraries and frameworks specifically designed for concurrent programming can effectively leverage these modern looping techniques to create highly performant and scalable applications.
For example, Python's multiprocessing
library can effectively parallelize operations on iterable objects, significantly reducing execution time for computationally intensive tasks. Similar capabilities exist in other languages like Java and C++, allowing for improved performance through concurrent iteration.
Risks and Mitigations in Modern Looping
While modern looping techniques offer many advantages, it's important to consider potential risks. Overuse of higher-order functions, for instance, can sometimes lead to less readable code if not implemented carefully. Additionally, incorrect use of iterators can result in unexpected behavior or performance issues. Thorough testing and careful consideration of code complexity are essential to mitigate these risks.
Impact and Implications of Modern Looping
The adoption of modern looping techniques has profound implications for software development. It promotes cleaner, more readable, and maintainable code, leading to reduced development time and improved software quality. The enhanced performance offered by concurrent iteration can significantly impact the efficiency of applications, especially those dealing with large datasets or computationally intensive tasks. Moreover, the move towards more functional programming paradigms, enabled by higher-order functions for iteration, contributes to improved code reliability and scalability.
Deep Dive into Iterators: A Case Study in Python
Python's iterator protocol allows for flexible and efficient iteration over various data structures. An iterator object must implement two special methods: __iter__()
(which returns the iterator object itself) and __next__()
(which returns the next item in the sequence or raises a StopIteration
exception when the end is reached).
Consider a custom iterator for generating Fibonacci numbers:
class FibonacciIterator:
def __init__(self, max_value):
self.a, self.b = 0, 1
self.max_value = max_value
def __iter__(self):
return self
def __next__(self):
if self.a > self.max_value:
raise StopIteration
result = self.a
self.a, self.b = self.b, self.a + self.b
return result
fib_iterator = FibonacciIterator(100)
for num in fib_iterator:
print(num)
This example showcases how a custom iterator can be created to generate a sequence based on specific logic. This level of flexibility is a key advantage of using iterators for iteration.
Frequently Asked Questions (FAQ)
Q1: What are the main benefits of using modern looping techniques?
A1: Modern looping techniques offer improved code readability, maintainability, efficiency (especially with concurrent processing), and often lead to more concise code, reducing development time and improving overall software quality.
Q2: Are traditional for
and while
loops obsolete?
A2: No, traditional loops remain valuable for simple iteration tasks. However, for more complex scenarios, modern techniques often offer superior solutions in terms of readability and efficiency. The choice depends on the specific context.
Q3: How do I choose between using iterators and higher-order functions?
A3: Iterators are best suited for situations where you need fine-grained control over the iteration process, especially when dealing with custom data structures or complex sequences. Higher-order functions excel at expressing iterative operations concisely and declaratively, often for tasks involving transformations or filtering of data.
Q4: What are some common pitfalls to avoid when using modern looping techniques?
A4: Overly complex higher-order function chains can reduce readability. Improper handling of iterators (e.g., infinite loops) can lead to errors. Always test your code thoroughly and prioritize clear, maintainable code.
Q5: Can modern loops enhance the performance of my applications?
A5: Yes, especially when coupled with concurrent programming techniques. Modern loops can significantly improve performance for computationally intensive tasks, particularly those involving large datasets or parallelizable operations.
Q6: Are there specific language features I should be aware of when using modern loops?
A6: Each programming language has its own approaches to modern looping. Familiarity with built-in functions like map
, filter
, reduce
(in languages that support them), and understanding of iterator protocols are crucial for effective implementation.
Actionable Tips for Mastering Modern Loops
- Embrace iterators: Learn how to use iterators in your preferred language to efficiently handle sequences and custom data structures.
- Master higher-order functions: Familiarize yourself with
map
,filter
,reduce
, and other relevant functions to express iteration concisely. - Prioritize readability: Choose the looping technique that makes your code the most readable and maintainable. Avoid excessive nesting or overly complex expressions.
- Handle exceptions gracefully: Implement robust error handling to prevent crashes and ensure application stability.
- Consider concurrency: Explore concurrent programming techniques to enhance the performance of your iterative operations.
- Use generators for memory efficiency: For large datasets, generators can avoid loading everything into memory simultaneously, leading to better performance and lower memory consumption.
Conclusion
Modern looping techniques represent a significant advancement in programming practices. By embracing these techniques, developers can create more efficient, readable, and maintainable code. The benefits extend beyond simple code improvements; the capacity for concurrency offered by modern loops unlocks new possibilities for high-performance and scalable applications. Understanding and effectively utilizing these techniques is crucial for any developer striving to write high-quality, robust, and efficient software. The continued evolution of programming languages and paradigms suggests that the future of iteration will likely involve even more sophisticated and powerful looping mechanisms.
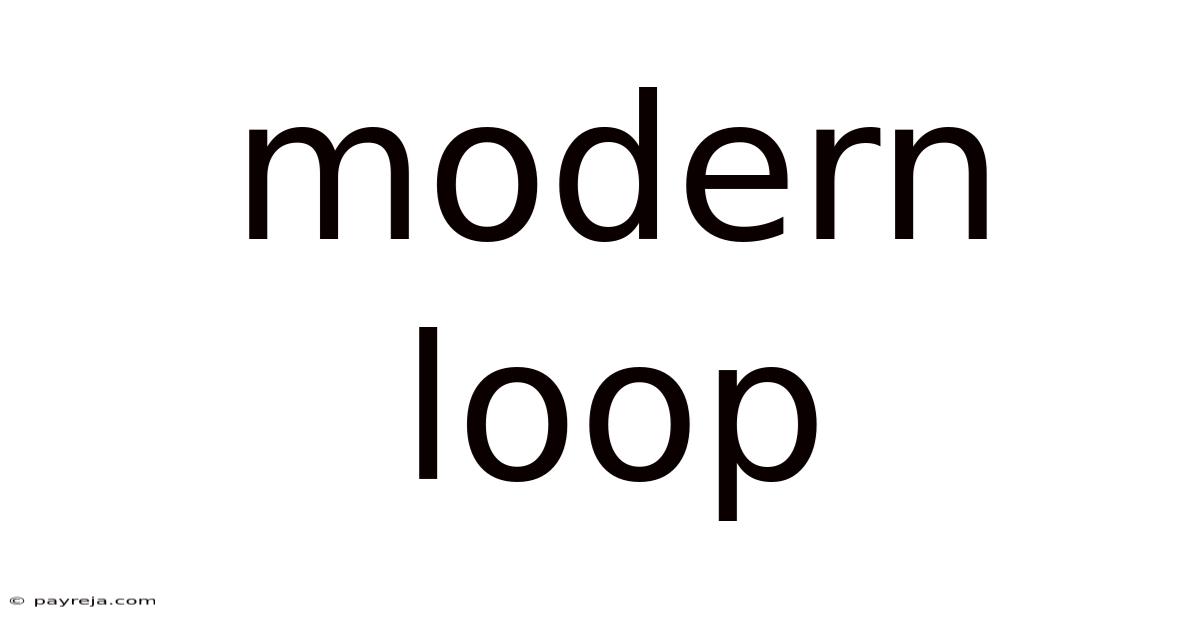
Thank you for visiting our website wich cover about Modern Loop. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Also read the following articles
Article Title | Date |
---|---|
Lightspeed Crm Integration | Mar 16, 2025 |
Microsoft Dynamics Crm Remote Jobs | Mar 16, 2025 |
Tforce Near Me | Mar 16, 2025 |
Electronic Customer Relationship Management Journal | Mar 16, 2025 |
Electronic Customer Relationship Management Meaning | Mar 16, 2025 |