How To Build Crm With Python
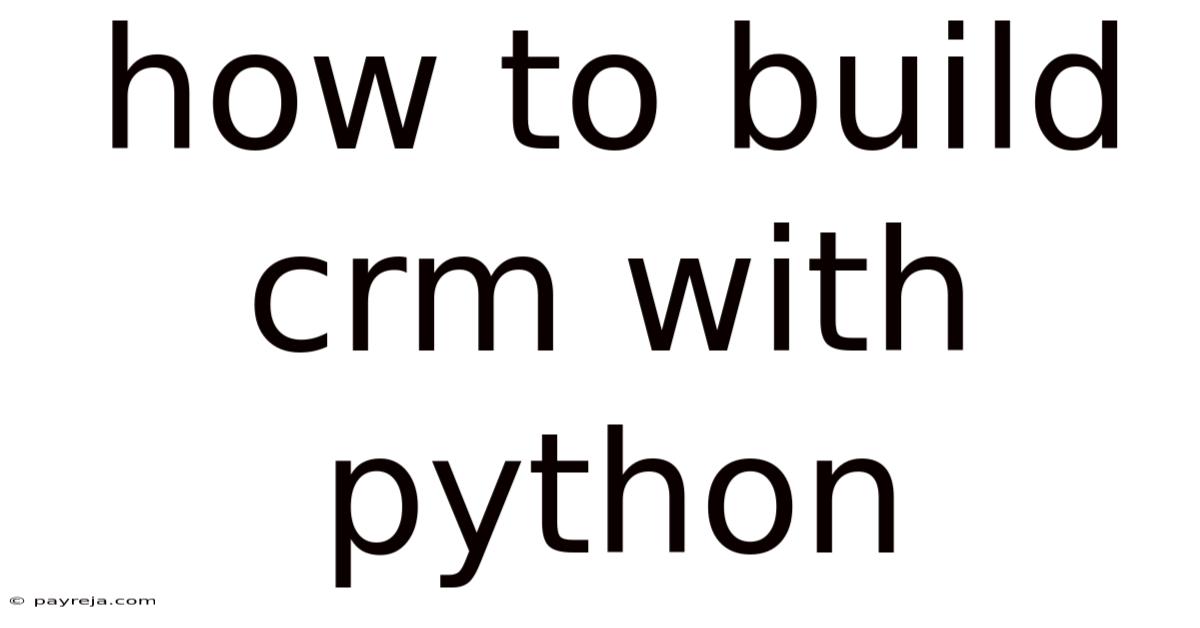
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit Best Website meltwatermedia.ca. Don't miss out!
Table of Contents
Building a CRM with Python: A Comprehensive Guide
Unlocking the power of Python to build a customized CRM system offers unparalleled flexibility and control.
Editor’s Note: This article on building a CRM with Python was published today, providing you with the latest insights and best practices.
Why build a CRM with Python? In today's competitive landscape, efficient customer relationship management (CRM) is paramount. While pre-built CRM solutions exist, they often lack the customization needed to perfectly align with a specific business's needs and workflow. Building a CRM using Python offers the flexibility to tailor the system to your unique requirements, integrate seamlessly with existing tools, and leverage the vast Python ecosystem for powerful features. This approach empowers businesses of all sizes to gain a competitive edge through efficient data management, improved customer interaction, and enhanced sales processes. This article explores the various aspects of creating a robust CRM using Python, from database selection to user interface design.
This article covers the key steps in building a Python-based CRM, including database design, API integration, user interface development, and deployment strategies. Readers will gain a practical understanding of the process and the ability to adapt these techniques to their own business needs. You’ll learn how to leverage Python’s power and flexibility to create a custom CRM solution that perfectly fits your business requirements.
Choosing the Right Tools
The foundation of any successful CRM lies in the selection of appropriate tools and technologies. For a Python-based CRM, several key components need careful consideration:
-
Database: The database is the heart of the CRM, storing all customer data. Popular choices include:
- PostgreSQL: A powerful, open-source relational database known for its scalability and reliability. Excellent for large datasets and complex relationships.
- MySQL: Another widely used open-source relational database, offering good performance and ease of use. A strong contender for smaller to medium-sized businesses.
- SQLite: A lightweight, file-based database ideal for smaller applications or prototyping. Not recommended for large-scale, high-traffic CRMs.
-
Web Framework: A web framework simplifies the development of the user interface and backend logic. Popular Python frameworks include:
- Django: A high-level framework offering a robust structure and built-in features like authentication and ORM (Object-Relational Mapper). Excellent for complex applications but can have a steeper learning curve.
- Flask: A lightweight and flexible microframework, ideal for smaller projects or when greater control over the application's architecture is desired. Offers a gentle learning curve and strong community support.
-
ORM (Object-Relational Mapper): An ORM translates data between the programming language (Python) and the database. Django includes its own ORM, while Flask often uses ORMs like SQLAlchemy, which offers greater flexibility.
-
UI Framework: The user interface is crucial for user experience. Options include:
- React (with Python backend): A JavaScript library allowing for dynamic and responsive user interfaces. Requires knowledge of JavaScript and React but delivers a modern user experience.
- HTML, CSS, and JavaScript (with Python backend): A classic approach offering direct control over the UI. Suitable for developers with strong front-end skills.
-
API Integration: Consider integrating with other services, like email marketing platforms (Mailchimp, SendGrid), payment gateways (Stripe, PayPal), and calendar applications (Google Calendar). Python's rich library ecosystem makes this relatively straightforward.
Database Design: The Blueprint of Your CRM
Before writing a single line of code, a well-defined database schema is essential. This schema outlines the tables, fields, and relationships within your CRM database. Here's a sample schema:
Table Name | Column Name | Data Type | Constraints |
---|---|---|---|
customers |
id |
INTEGER | PRIMARY KEY, AUTOINCREMENT |
first_name |
VARCHAR(255) | NOT NULL | |
last_name |
VARCHAR(255) | NOT NULL | |
email |
VARCHAR(255) | UNIQUE, NOT NULL | |
phone |
VARCHAR(20) | ||
company |
VARCHAR(255) | ||
address |
TEXT | ||
contacts |
id |
INTEGER | PRIMARY KEY, AUTOINCREMENT |
customer_id |
INTEGER | FOREIGN KEY (customers .id ) |
|
contact_name |
VARCHAR(255) | NOT NULL | |
contact_email |
VARCHAR(255) | ||
contact_phone |
VARCHAR(20) | ||
interactions |
id |
INTEGER | PRIMARY KEY, AUTOINCREMENT |
customer_id |
INTEGER | FOREIGN KEY (customers .id ) |
|
contact_id |
INTEGER | FOREIGN KEY (contacts .id ) |
|
interaction_type |
VARCHAR(50) | ('email', 'phone', 'meeting', 'other') | |
interaction_date |
DATETIME | NOT NULL | |
notes |
TEXT |
This is a simplified example. A real-world CRM might require additional tables for sales opportunities, tasks, deals, products, and other relevant information. Consider data normalization to avoid redundancy and improve data integrity.
Building the Backend (Using Flask and SQLAlchemy)
This section outlines a basic Flask backend with SQLAlchemy for database interaction. This example focuses on core CRM functionality: adding and retrieving customer data.
from flask import Flask, request, jsonify
from flask_sqlalchemy import SQLAlchemy
from sqlalchemy import create_engine, text
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'postgresql://user:password@localhost/crm_db' # Replace with your database credentials
app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False
db = SQLAlchemy(app)
class Customer(db.Model):
id = db.Column(db.Integer, primary_key=True)
first_name = db.Column(db.String(255), nullable=False)
last_name = db.Column(db.String(255), nullable=False)
email = db.Column(db.String(255), unique=True, nullable=False)
# ... other columns ...
def to_dict(self):
return {
'id': self.id,
'first_name': self.first_name,
'last_name': self.last_name,
'email': self.email,
# ... other columns ...
}
@app.route('/customers', methods=['POST'])
def add_customer():
data = request.get_json()
new_customer = Customer(**data)
db.session.add(new_customer)
db.session.commit()
return jsonify({'message': 'Customer added successfully', 'customer': new_customer.to_dict()}), 201
@app.route('/customers', methods=['GET'])
def get_customers():
customers = Customer.query.all()
return jsonify([customer.to_dict() for customer in customers])
if __name__ == '__main__':
db.create_all()
app.run(debug=True)
This code demonstrates basic CRUD (Create, Read, Update, Delete) operations for customers. Error handling and input validation are crucial aspects to be added in a production environment.
Developing the User Interface
The UI significantly impacts user experience. Consider using a framework like React for a dynamic and modern interface, or sticking with HTML, CSS, and JavaScript for greater control. The choice depends on your development skills and project requirements. The UI would include forms for adding/editing customers, displaying customer lists, filtering/searching capabilities, and potentially dashboards for visualizing key metrics.
API Integration: Expanding Functionality
Integrating with external services enhances your CRM's capabilities. For instance:
- Email Marketing: Use the Mailchimp API to send automated emails based on customer interactions.
- Payment Gateways: Integrate Stripe or PayPal for online payments processing.
- Calendar Applications: Connect with Google Calendar to schedule meetings and track appointments.
Python's extensive library support simplifies these integrations. Many services offer well-documented APIs and Python client libraries.
Deployment and Scaling
Deploying your CRM depends on your needs and resources. Options include:
- Cloud Platforms: AWS, Google Cloud, or Azure offer scalability and reliability.
- Containerization (Docker): Package your application and dependencies into containers for easier deployment and portability.
- Serverless Functions: Use serverless platforms like AWS Lambda or Google Cloud Functions for event-driven architectures.
Scaling your CRM requires considering database scaling (sharding, read replicas), load balancing, and caching strategies.
Key Takeaways: Building Your Python CRM
Feature | Description | Importance |
---|---|---|
Database Selection | Choose PostgreSQL, MySQL, or SQLite based on scale and complexity. | Data storage, performance, and scalability |
Web Framework | Django or Flask provide structure and simplify development. | Backend development efficiency |
ORM | SQLAlchemy or Django ORM map Python objects to database tables. | Efficient database interaction |
UI Framework | React, or HTML/CSS/JavaScript offer different levels of complexity and control. | User experience and application interactivity |
API Integration | Connect to email marketing, payment gateways, and other services. | Expanded functionality and automation |
Deployment Strategy | Cloud platforms, Docker, or serverless functions offer different options. | Scalability, reliability, and maintainability |
The Connection Between Data Security and CRM Development
Data security is paramount when building a CRM. Protecting sensitive customer information requires careful consideration throughout the development process:
Roles and Real-World Examples:
- Data Encryption: Encrypt data both at rest (in the database) and in transit (between the client and server). Libraries like cryptography provide tools for encryption.
- Access Control: Implement robust access control mechanisms to restrict access to sensitive data based on user roles. Django and Flask offer built-in authentication and authorization features.
- Input Validation: Sanitize user input to prevent SQL injection and cross-site scripting (XSS) attacks. Libraries like WTForms can help with this.
Risks and Mitigations:
- SQL Injection: Protect against SQL injection attacks by using parameterized queries or ORMs that handle escaping automatically.
- Cross-Site Scripting (XSS): Escape user-provided data to prevent XSS vulnerabilities.
- Data Breaches: Implement strong security measures, including regular security audits and penetration testing, to minimize the risk of data breaches.
Impact and Implications:
A secure CRM protects your business from financial loss, reputational damage, and legal repercussions. Ignoring data security can have severe consequences. Investing in robust security measures is essential for building a trustworthy and reliable CRM.
Diving Deeper into Data Security Best Practices
Data security is a multifaceted issue. Beyond the basics, consider these advanced techniques:
- Two-Factor Authentication (2FA): Add an extra layer of security by requiring users to provide a second form of authentication (e.g., a code from a mobile app).
- Regular Security Audits: Conduct regular security audits to identify vulnerabilities and weaknesses.
- Intrusion Detection and Prevention Systems (IDPS): Implement IDPS to monitor network traffic and detect malicious activity.
- Data Loss Prevention (DLP): Use DLP tools to prevent sensitive data from leaving the organization's network.
These advanced techniques help create a more secure CRM system, protecting customer data from unauthorized access and breaches.
Frequently Asked Questions (FAQs)
-
Q: What's the best database for a small CRM? A: SQLite is suitable for very small projects, but MySQL or PostgreSQL are better for scalability as your business grows.
-
Q: How difficult is it to learn Python for CRM development? A: Python is known for its readability and ease of use, making it relatively accessible for beginners.
-
Q: Can I integrate my CRM with existing software? A: Yes, Python makes integrating with various APIs straightforward.
-
Q: What are the deployment options for a Python CRM? A: You can deploy on cloud platforms, using Docker containers, or serverless functions.
-
Q: How much does it cost to build a Python CRM? A: Cost depends on complexity, features, and development time. Open-source components reduce costs, but skilled developers are necessary.
-
Q: Is it better to build or buy a CRM? A: Building offers customization, but buying provides a readily available solution. The best choice depends on your specific needs and resources.
Actionable Tips for Building Your Python CRM
-
Start Small: Begin with a minimal viable product (MVP) and add features iteratively.
-
Prioritize Security: Implement security measures from the outset.
-
Use Version Control (Git): Track code changes and collaborate effectively.
-
Test Thoroughly: Write unit tests and integration tests to ensure code quality.
-
Document Your Code: Create clear documentation to aid maintenance and future development.
-
Choose the Right Tools: Select tools based on project size, experience, and long-term goals.
Conclusion
Building a CRM with Python offers unparalleled flexibility and control. By carefully selecting the right tools, designing a robust database, and implementing effective security measures, businesses can create a customized CRM that perfectly aligns with their specific needs. Remember that building a successful CRM is an iterative process that requires planning, development, testing, and ongoing maintenance. Understanding the core concepts discussed in this article provides a solid foundation for building your own powerful and customized CRM solution. The ability to tailor the system to your specific business needs, integrate with existing systems seamlessly, and leverage Python's powerful capabilities make this a worthwhile endeavor for businesses looking for a competitive advantage. Remember to prioritize data security and scalability to ensure your CRM remains reliable and efficient as your business grows.
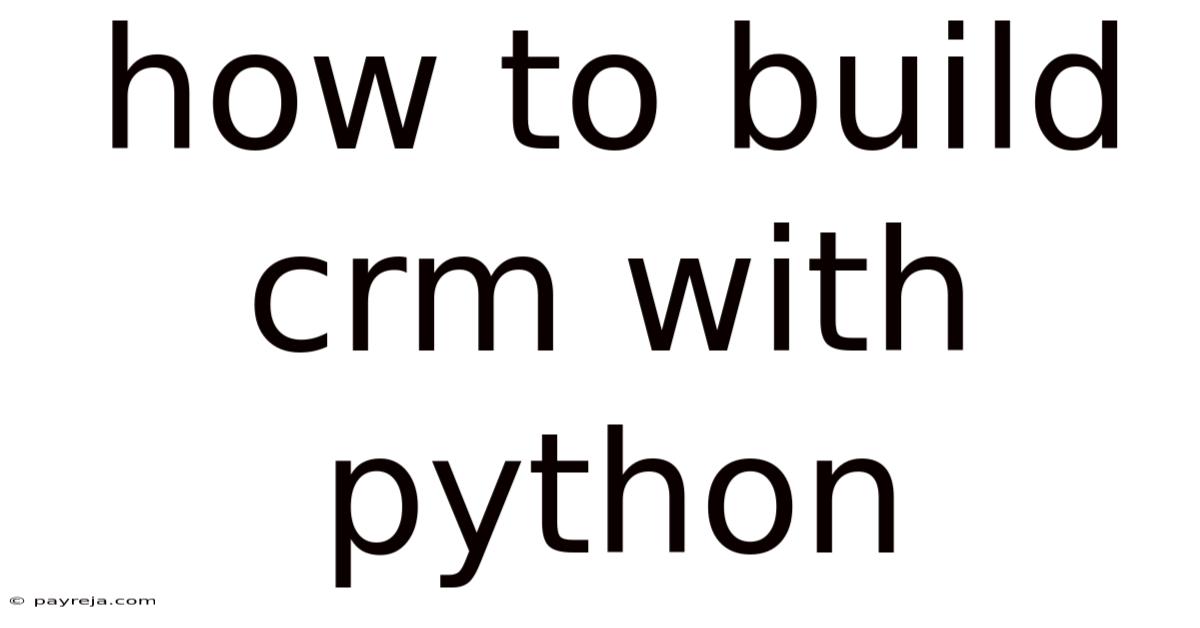
Thank you for visiting our website wich cover about How To Build Crm With Python. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Also read the following articles
Article Title | Date |
---|---|
What Does The Acronym Crm Stand For In The Context Of Customer Support | Apr 20, 2025 |
Best Crm For Real Estate | Apr 20, 2025 |
Is Slack A Crm System | Apr 20, 2025 |
Bullhorn Crm Reviews | Apr 20, 2025 |
How To Use Outlook Like A Crm | Apr 20, 2025 |