Dynamo Crud
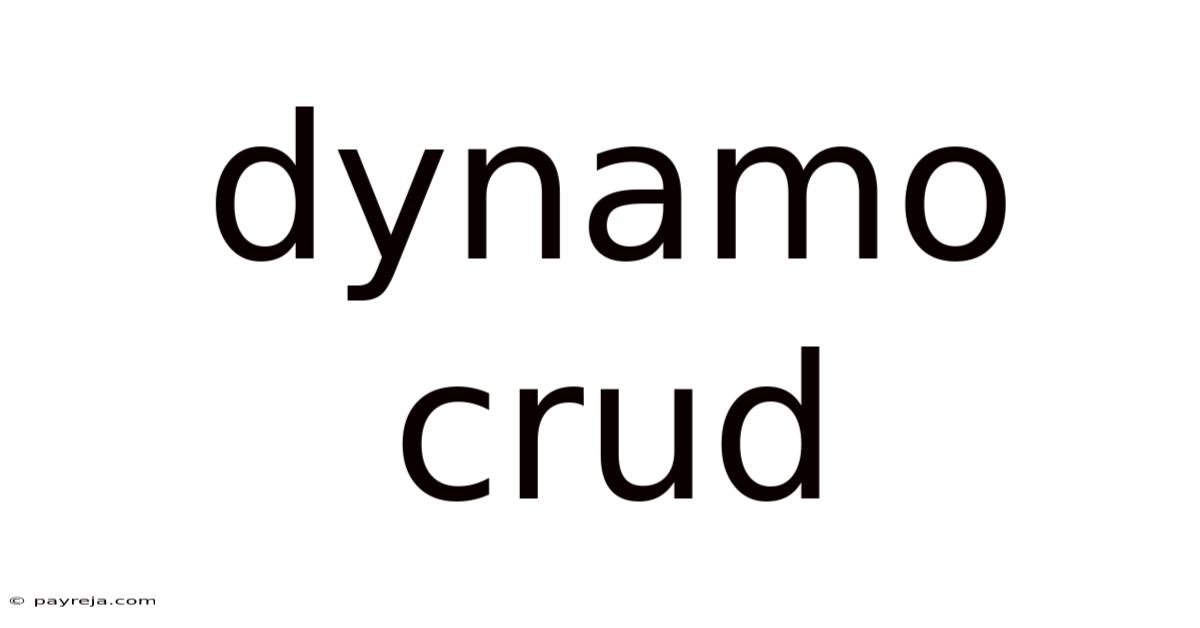
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit Best Website meltwatermedia.ca. Don't miss out!
Table of Contents
DynamoDB CRUD Operations: A Deep Dive into Efficient Data Management
Unlocking the power of DynamoDB's flexibility could revolutionize your data management.
Editor’s Note: This article on DynamoDB CRUD operations has been published today, providing you with the most up-to-date information and best practices.
DynamoDB, Amazon's fully managed NoSQL database service, offers unparalleled scalability and performance. Understanding its Create, Read, Update, and Delete (CRUD) operations is crucial for leveraging its full potential. This article delves into the intricacies of DynamoDB CRUD, providing a comprehensive guide for developers of all skill levels. We will explore best practices, potential pitfalls, and practical applications across various industries. The ability to efficiently manage data within DynamoDB is key to building robust and scalable applications.
What You Will Learn:
This article covers the fundamental CRUD operations within DynamoDB, exploring efficient query strategies, data modeling techniques, and best practices for error handling. You'll gain actionable insights to optimize your application's performance and scalability, along with a clear understanding of when and how to apply different DynamoDB features for optimal results.
Understanding DynamoDB's Data Model
Before diving into CRUD operations, understanding DynamoDB's schema-less nature is vital. Unlike relational databases, DynamoDB doesn't enforce a rigid schema. Data is organized into tables, each containing items. An item is a collection of attribute-value pairs, similar to a JSON object or a key-value store. Each item is uniquely identified by a primary key, which can be a single attribute (partition key) or a composite key (partition key and sort key). This flexible schema allows for rapid development and adaptation to evolving data needs.
DynamoDB CRUD Operations: A Detailed Exploration
1. Create (Inserting Data):
Creating items in DynamoDB involves specifying the primary key and other attributes. The PutItem
API call is used for this purpose. It's important to ensure the primary key is unique within the table; otherwise, the operation will fail.
Example (using AWS SDK for JavaScript):
const params = {
TableName: 'MyTable',
Item: {
'userId': {S: 'user123'}, // Partition key (String type)
'userName': {S: 'John Doe'},
'email': {S: '[email protected]'}
}
};
docClient.put(params, function(err, data) {
if (err) {
console.error("Unable to add item. Error JSON:", JSON.stringify(err, null, 2));
} else {
console.log("Added item:", JSON.stringify(data, null, 2));
}
});
This example demonstrates inserting a new item with a partition key (userId
). Note the use of attribute value types (e.g., S
for String). Proper data type handling is crucial for efficient querying and data retrieval.
2. Read (Retrieving Data):
Retrieving data from DynamoDB relies on the primary key. The GetItem
API call retrieves a single item based on its primary key. For retrieving multiple items, Query
and Scan
are used, but these are less efficient for large datasets and should be optimized using appropriate filtering and pagination.
Example (using AWS SDK for JavaScript - GetItem):
const params = {
TableName: 'MyTable',
Key: {
'userId': {S: 'user123'}
}
};
docClient.get(params, function(err, data) {
if (err) {
console.error("Unable to read item. Error JSON:", JSON.stringify(err, null, 2));
} else {
console.log("GetItem succeeded:", JSON.stringify(data, null, 2));
}
});
This retrieves the item with userId: 'user123'
. Query
and Scan
provide more complex retrieval capabilities, enabling filtering and sorting based on attributes other than the primary key.
3. Update (Modifying Data):
Updating items involves modifying existing attributes. The UpdateItem
API call is used for this. It allows for conditional updates using ConditionExpression
to prevent unintended overwrites.
Example (using AWS SDK for JavaScript - UpdateItem):
const params = {
TableName: 'MyTable',
Key: {
'userId': {S: 'user123'}
},
UpdateExpression: "SET email = :newEmail",
ExpressionAttributeValues: {
':newEmail': {S: '[email protected]'}
},
ReturnValues: "UPDATED_NEW"
};
docClient.update(params, function(err, data) {
if (err) {
console.error("Unable to update item. Error JSON:", JSON.stringify(err, null, 2));
} else {
console.log("UpdateItem succeeded:", JSON.stringify(data, null, 2));
}
});
This example updates the email
attribute. UpdateExpression
and ExpressionAttributeValues
are used to specify the update operation and the new value. ReturnValues
controls what data is returned after the update.
4. Delete (Removing Data):
Deleting items from DynamoDB uses the DeleteItem
API call. Similar to updates, conditional deletes can be implemented using ConditionExpression
to ensure data integrity.
Example (using AWS SDK for JavaScript - DeleteItem):
const params = {
TableName: 'MyTable',
Key: {
'userId': {S: 'user123'}
}
};
docClient.delete(params, function(err, data) {
if (err) {
console.error("Unable to delete item. Error JSON:", JSON.stringify(err, null, 2));
} else {
console.log("DeleteItem succeeded:", JSON.stringify(data, null, 2));
}
});
This deletes the item with userId: 'user123'
.
Best Practices for DynamoDB CRUD Operations
- Efficient Data Modeling: Choose appropriate primary key structures to optimize query performance. Consider the access patterns of your application when designing your tables.
- Consistent Data Types: Maintain consistency in data types for attributes within an item. Inconsistent types can lead to query errors and performance issues.
- Conditional Updates and Deletes: Use
ConditionExpression
to prevent unintended data modifications and ensure data integrity. - Batch Operations: Utilize
BatchWriteItem
andBatchGetItem
for improved efficiency when working with multiple items. - Pagination: Implement pagination for
Query
andScan
operations to handle large datasets effectively. - Error Handling: Implement robust error handling to gracefully manage potential issues, such as conflicts and throttling.
- Capacity Planning: Understand and manage your provisioned or on-demand throughput capacity to ensure optimal performance.
The Connection Between Data Modeling and DynamoDB CRUD Efficiency
The choice of primary key significantly influences the efficiency of DynamoDB CRUD operations. A poorly designed primary key can lead to full table scans (Scan
), which are significantly less efficient than targeted queries (Query
). For instance, if frequently querying based on a specific attribute, that attribute should be considered for inclusion in the primary key. Careful consideration of access patterns, anticipated query types, and data distribution is crucial for efficient data modeling in DynamoDB.
Roles and Real-World Examples
- E-commerce: Managing product catalogs, user profiles, and order information. The primary key could be
productId
for product information anduserId
for user profiles. - Social Media: Storing user posts, comments, and likes. The primary key could be a composite key combining
userId
andpostId
. - Gaming: Tracking player progress, inventory, and game statistics. The primary key could be
userId
combined with agameSessionId
for tracking in-game activities.
Risks and Mitigations
- Incorrect Data Modeling: Leads to inefficient queries and high operational costs. Mitigate by carefully analyzing access patterns and choosing appropriate primary keys.
- Insufficient Provisioned Throughput: Results in throttling and performance degradation. Mitigate by monitoring performance metrics and adjusting throughput capacity as needed.
- Data Consistency Issues: Without proper conditional updates and deletes, data inconsistencies can occur. Mitigate by using
ConditionExpression
and implementing appropriate locking mechanisms when necessary.
Impact and Implications
Efficient DynamoDB CRUD operations directly impact application performance, scalability, and operational costs. Well-structured data models and efficient query strategies can significantly reduce latency, improve response times, and minimize costs associated with data storage and retrieval.
Deeper Dive into Data Modeling
The most impactful aspect of DynamoDB performance is data modeling. A well-designed schema can mean the difference between blazing-fast queries and crippling slowdowns. The critical consideration is how frequently you’ll need to access data based on various attributes.
Attribute | Frequency of Access | Primary Key Consideration |
---|---|---|
User ID | Very High | Partition Key |
Product Category | High | Partition Key or Sort Key |
Order Date | Medium | Sort Key |
Product Name | Low | Secondary Index (GSI) |
Customer Location | Medium | Secondary Index (GSI) |
Global Secondary Indexes (GSIs) are particularly important when frequently querying based on non-primary key attributes. GSIs provide additional access paths to your data, enabling faster queries without full table scans. However, GSIs introduce additional operational costs, so careful consideration is necessary.
Frequently Asked Questions (FAQ)
-
What is the difference between
Query
andScan
in DynamoDB?Query
uses the primary key (or a secondary index) for efficient data retrieval.Scan
scans the entire table, significantly less efficient for large datasets. -
How do I handle concurrent updates in DynamoDB? Use
ConditionExpression
withUpdateItem
orDeleteItem
to ensure only the latest version of the data is updated. -
What are the best practices for choosing a primary key? Consider the most frequent access patterns and choose attributes that best suit those patterns. Consider using a composite key if necessary.
-
How can I optimize my DynamoDB queries for performance? Use appropriate filters, pagination, and consider GSIs for frequent queries on non-primary key attributes.
-
What are the different data types supported in DynamoDB? DynamoDB supports various data types including String, Number, Binary, Boolean, List, Map, and Null. Choosing the correct data type is important for query optimization.
-
How do I monitor the performance of my DynamoDB tables? Use Amazon CloudWatch to monitor metrics such as consumed capacity, throttled requests, and latency.
Actionable Tips for DynamoDB CRUD Optimization
-
Analyze Access Patterns: Before designing your schema, carefully analyze how your application will interact with the data. Identify the most frequent queries and access patterns.
-
Choose the Right Primary Key: Design a primary key that optimizes for your most frequent access patterns. Consider using a composite key if your data has multiple dimensions of access.
-
Leverage Global Secondary Indexes (GSIs): If you frequently query on attributes not part of the primary key, create GSIs to dramatically improve query performance.
-
Utilize Batch Operations: For bulk inserts, updates, or deletes, use
BatchWriteItem
andBatchGetItem
for efficiency. -
Implement Pagination: For large datasets, use pagination to avoid retrieving excessive data at once, improving response times.
-
Monitor Performance and Adjust Capacity: Regularly monitor your DynamoDB performance metrics using Amazon CloudWatch and adjust your provisioned throughput accordingly.
-
Conditional Updates and Deletes: Always use conditional updates and deletes to maintain data consistency and prevent conflicts.
-
Employ Efficient Query Strategies: Make sure to utilize filters (
FilterExpression
) to narrow down results, avoiding unnecessary data retrieval.
Conclusion
Mastering DynamoDB CRUD operations is essential for building scalable and efficient applications. By understanding the nuances of data modeling, utilizing appropriate API calls, and following best practices, developers can significantly optimize the performance and cost-effectiveness of their DynamoDB deployments. The flexibility and scalability of DynamoDB make it a powerful tool for a wide range of applications, but its efficiency relies heavily on informed design choices and a deep understanding of its capabilities. Continuous monitoring and optimization are crucial to ensure sustained performance and cost-effectiveness. Remember, a well-designed data model and strategic use of DynamoDB's features are the keys to unlocking its full potential.
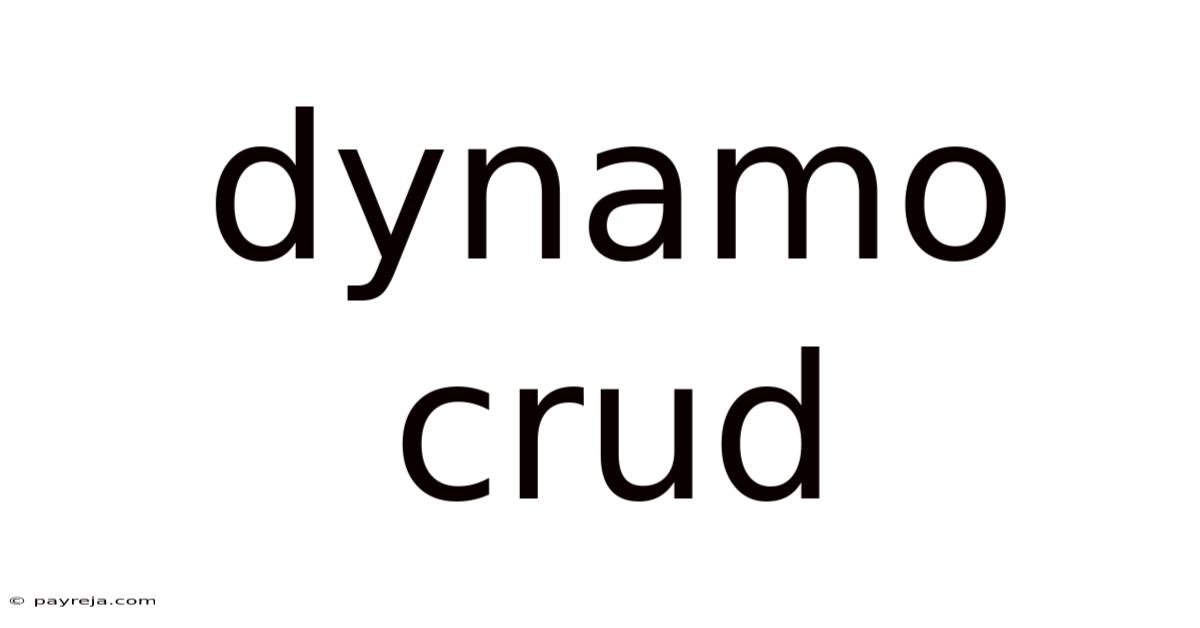
Thank you for visiting our website wich cover about Dynamo Crud. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Also read the following articles
Article Title | Date |
---|---|
Health Insurance Agency Crm | Apr 26, 2025 |
Luxor Play | Apr 26, 2025 |
What Is Relationship Management In Leadership | Apr 26, 2025 |
Major Companies In Mn | Apr 26, 2025 |
Hop Kalcer | Apr 26, 2025 |